The Underdog API allows you to mint, manage, & update your digital assets. We support compressed NFTs, Core NFTs, and fungible tokens.
Configuration
To get started with the Underdog API, you'll need to set up two pieces of configuration.
- Underdog API Endpoint
- API Key
Underdog API Endpoint
The Underdog API has two endpoints that connect to either Solana Mainnet or Solana Devnet.
Network | API Endpoint |
---|---|
Solana Mainnet | https://mainnet.underdogprotocol.com |
Solana Devnet | https://devnet.underdogprotocol.com |
For this example, we'll be using the Solana Devnet API endpoint. The Solana Mainnet API requires activating a paid Underdog plan.
API Key
You can get your API Key from the Underdog Dashboard. From the home page, head to Developers then hit Generate API Key. API Keys are only shown once so store it in a safe place.
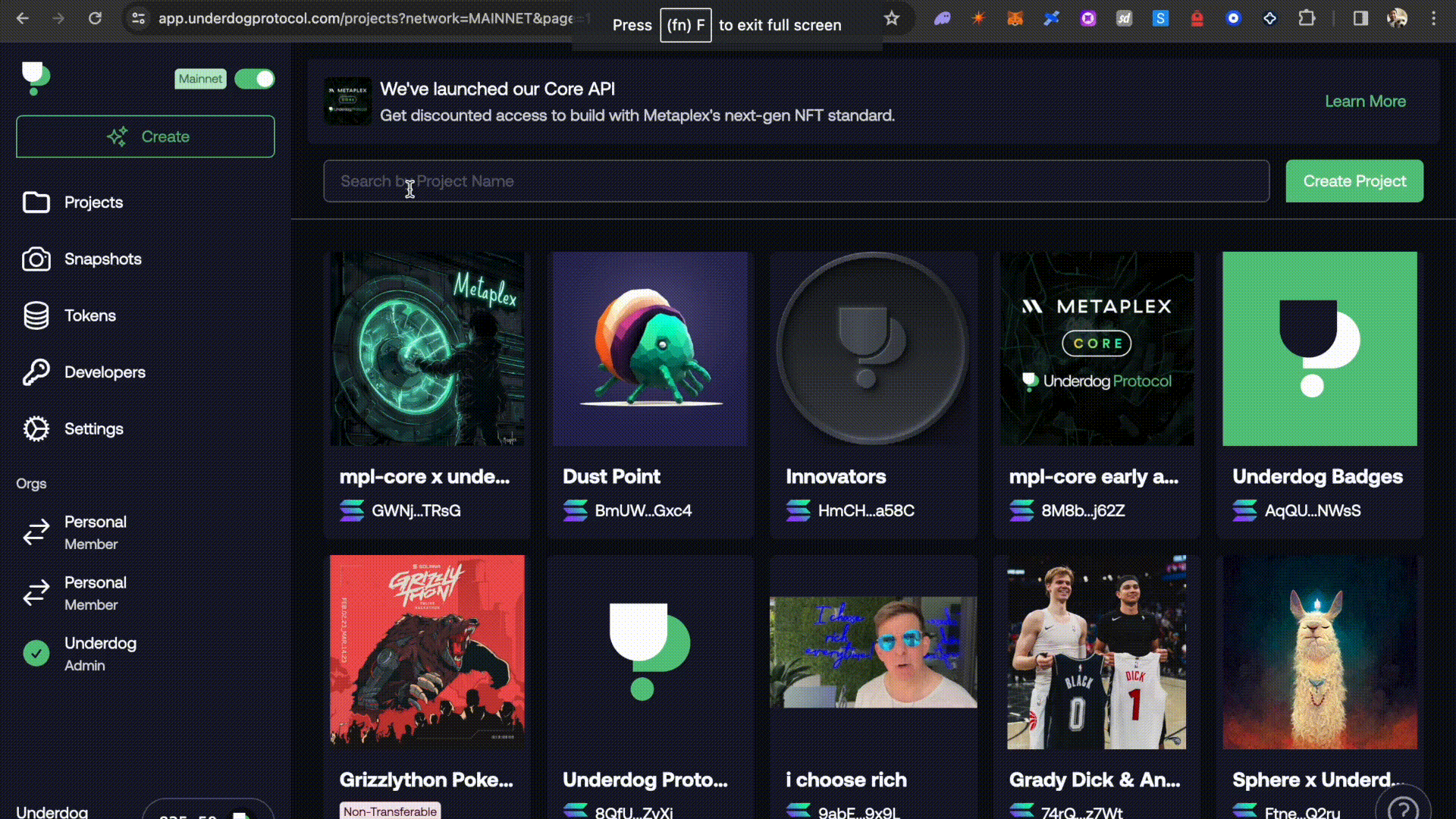
Complete Configuration
Now that you have an API endpoint and API key, we can start making requests to the Underdog API. Let's create a reusable axios
instance for the rest of this example.
const underdog = await axios.create({
baseURL: "https://devnet.underdogprotocol.com",
headers: {
Authorization: `Bearer 3e5bd4509a22fb.885cc54a33e24d398ce8bdd3c2092786`,
},
});
Create a Project
Finally, some on-chain interaction. Once you have your configuration set up, you can Create a Project which handles creating the collection NFT, securing it in a secure vault, and making sure all your NFTs are verified as part of the collection.
const createProjectResponse = await underdog.post(`/v2/projects`, {
name: "Underdog Project",
image: "https://i.imgur.com/jMHjy8x.jpeg",
});
Create an NFT
Time to Create an NFT. You can grab the Project's ID from the API response, a human-readable identifier for your Project and then pass in the metadata for your NFT.
const createNftResponse = await underdog.post(`/v2/projects/${createProjectResponse.data.projectId}/nfts`, {
name: "Underdog #1",
image: "https://i.imgur.com/jMHjy8x.jpeg",
});
Full Code
Want to just copy-paste the full code and run it yourself?
import axios from "axios";
export const main = async () => {
const underdog = await axios.create({
baseURL: "https://devnet.underdogprotocol.com",
headers: {
Authorization: `Bearer 3e5bd4509a22fb.885cc54a33e24d398ce8bdd3c2092786`,
},
});
const createProjectResponse = await underdog.post(`/v2/projects`, {
name: "Underdog Project",
image: "https://i.imgur.com/jMHjy8x.jpeg",
});
const createNftResponse = await underdog.post(`/v2/projects/${createProjectResponse.data.projectId}/nfts`, {
name: "Underdog #1",
image: "https://i.imgur.com/jMHjy8x.jpeg",
});
};